Java is one of the most popular programming languages, known for its platform independence, scalability, and robust ecosystem java. Whether you’re a novice developer or an experienced professional, mastering Java requires attention to detail and adherence to best practices. Here are 10 essential tips every Java developer should know to write clean, efficient, and maintainable code.
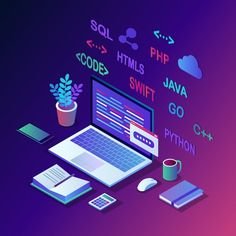
1. Follow Naming Conventions
Adhering to Java’s standard naming conventions ensures your code is readable and professional. Use CamelCase for classes (MyClass
) and variables/methods (myVariable
or myMethod
), and UPPERCASE_WITH_UNDERSCORES for constants (MAX_SIZE
).
javaCopy code// Example of proper naming
public class UserAccount {
private String userName;
private final int MAX_RETRIES = 3;
}
2. Use Meaningful Variable Names
Avoid single-character or ambiguous variable names unless used in a concise context, like a loop. Descriptive names make the code self-documenting and easier to understand.
javaCopy code// Bad practice
int x = 10;
// Good practice
int maxConnections = 10;
3. Leverage the Power of Java Collections
Avoid using arrays unless you have a specific reason. The Collections framework provides powerful tools like ArrayList
, HashMap
, and HashSet
for handling data efficiently.
javaCopy code// Example with ArrayList
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
4. Avoid Memory Leaks
Be cautious with resources like database connections, file streams, or objects in collections. Use try-with-resources and remove unused objects to prevent memory leaks.
javaCopy codetry (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
5. Write Immutable Classes When Appropriate
Immutable objects are thread-safe and reduce bugs in multi-threaded environments. Use the final
keyword for classes, fields, and methods when designing immutable classes.
javaCopy codepublic final class User {
private final String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
6. Use Streams for Cleaner Code
The Stream API introduced in Java 8 allows for more concise and readable code when processing collections or arrays.
javaCopy code// Example: Filtering a list
List<String> names = List.of("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
7. Avoid Using Raw Types
Generics ensure type safety and reduce the need for type casting, which can lead to runtime errors.
javaCopy code// Bad practice: Raw types
List items = new ArrayList();
items.add("item");
String item = (String) items.get(0);
// Good practice: Generics
List<String> items = new ArrayList<>();
items.add("item");
String item = items.get(0);
8. Optimize String Handling
Strings in Java are immutable. Use StringBuilder
or StringBuffer
for concatenation in loops to avoid creating unnecessary objects.
javaCopy code// Inefficient
String result = "";
for (int i = 0; i < 10; i++) {
result += i;
}
// Efficient
StringBuilder result = new StringBuilder();
for (int i = 0; i < 10; i++) {
result.append(i);
}
9. Master Exception Handling
Handle exceptions gracefully using specific catches and avoid generic catch(Exception e)
unless necessary. Log the error or take corrective actions instead of simply suppressing it.
javaCopy codetry {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero.");
}
10. Keep Your Dependencies Updated
Outdated libraries can pose security risks and lack performance improvements. Use tools like Maven or Gradle to manage dependencies and check for updates regularly.
xmlCopy code<!-- Example Maven dependency -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.22</version>
</dependency>
Conclusion
By following these tips, you can write Java code that is not only functional but also efficient, secure, and easy to maintain. Whether you’re building a small application or an enterprise-level system, these best practices will set you up for success.